DEADLOCK SITUATION
When two threads are waiting for each other to get the lock and stuck for infinite time.
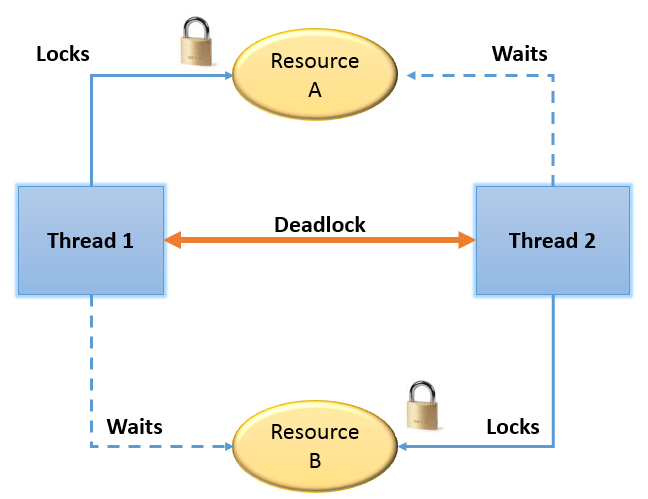
REASON BEHIND DEADLOCK
Multiple threads are not the real cause of deadlock, instead the way in which threads are requesting the lock.
HOW DEADLOCK HAPPENS ?
1. When using nested synchronized blocks
OR
2. When one synchronized method called from other
ANALYZING DEADLOCK
1. Take thread dump using kill -3
OR
2. Use jconsole
It will print which thread is locked on which object.
CODE TO REPRODUCE DEADLOCK
public void m1() {
synchronized(String.class) {
System.out.println("inside block for String");
synchronized(Double.class) {
System.out.println("inside block for Double");
}
}
}
public void m2() {
synchronized(Double.class) {
System.out.println("inside block for Double");
synchronized(String.class) {
System.out.println("inside block for String");
}
} }
FIXING OR AVOIDING DEADLOCK
- Provide ordered access to the object using synchronized blocks.
public void m1() {
synchronized(String.class) {
System.out.println("inside block for String");
synchronized(Double.class) {
System.out.println("inside block for Double");
}
}
}
public void m2() {
synchronized(String.class) {
System.out.println("inside block for String");
synchronized(Double.class) {
System.out.println("inside block for Double");
}
}
}
No comments:
Post a Comment
Note: only a member of this blog may post a comment.